Java Que. Bank Sem-4 BCA
- Hit Govani
- Apr 9, 2023
- 14 min read
Q. : 1) True or False
The size of char data-type is 1 byte in Java. ➡ FALSE
A single Java file many contain any number of class definitions. ➡ TRUE
Java source code can be written in files with any extension. ➡ FALSE
A Boolean value can be cast to an int. ➡ FALSE
Every class has at least one constructor available. ➡ TRUE
Static variable cannot be used in non-static methods. ➡ FALSE
Overloading of a constructor is not allowed in Java. ➡ FALSE
Java supports destructor. ➡ FALSE
String is a immutable object in java. ➡ TRUE
Object is an instance of a class. ➡ TRUE
The size of char data-type is 2 byte in java. ➡ TRUE
The default case is required in the switch selection structure. ➡ FALSE
“final” variables cannot be modified. ➡ TRUE
An abstract class must have at least one abstract method. ➡ FALSE
One class cannot be derived from more than one class in java. ➡ TRUE
The object created by String class can be modified. ➡ TRUE
Q. :2) Fill up the blank line
A method declared with final modifier cannot be overridden.
Java uses Unicode character set to represent characters.
It is possible to throw an exception explicitly using the C# throw or the Visual Basic Throw statement.
System Exception Class is the base class of all Exception class.
A package is a collection of classes and interfaces.
The setBackground() method sets the background color of an applet.
Method main is a public static method. Justify so that compiler can call it without the creation of an object or before the creation of an object of the class.
Q. :3) 2-3 Mark Questions
1. Draw compilation and interpretation process of java.
Ans. Java can be considered both a compiled and an interpreted language because its source code is first compiled into a binary byte-code. This byte-code runs on the Java Virtual Machine (JVM), which is usually a software-based interpreter.
2. What is the difference between method overloading and overriding in Java?
Ans. Overriding occurs when the method signature is the same in the superclass and the child class. Overloading occurs when two or more methods in the same class have the same name but different parameters.
3. What is the difference between final method and ordinary method of a class?
Ans. Static methods can be called by other static methods and only access the static members of the class. On the other hand, final methods cannot be overridden.
4. Explain the uses of keyword “super” and “extends” with code.
Ans. The super keyword refers to superclass (parent) objects. It is used to call superclass methods, and to access the superclass constructor. The most common use of the super keyword is to eliminate the confusion between superclasses and subclasses that have methods with the same name.
5. What is meant by interface? Explain with java code.
Ans. An interface is an abstract "class" that is used to group related methods with "empty" bodies: To access the interface methods, the interface must be "implemented" (kinda like inherited) by another class with the implements keyword (instead of extends ).
CODE:
interface Animal {
public void animalSound(); // interface method (does not have a body)
public void sleep(); // interface method (does not have a body)
}
class Pig implements Animal {
public void animalSound() {
System.out.println("The pig says: wee wee");
}
public void sleep() {
System.out.println("Zzz");
}
}
class Main {
public static void main(String[] args) {
Pig myPig = new Pig();
myPig.animalSound();
myPig.sleep();
}
}
6. How method overriding will be achieved in Java application?
Ans. In Java, method overriding occurs when a subclass (child class) has the same method as the parent class. In other words, method overriding occurs when a subclass provides a particular implementation of a method declared by one of its parent classes.
7. Explain the purpose of “Exception” class.
Ans. Exceptions provide the means to separate the details of what to do when something out of the ordinary happens from the main logic of a program. In traditional programming, error detection, reporting, and handling often lead to confusing spaghetti code.
8. Explain the uses of the keywords “throw” and “finally”.
Ans.
Java Throw:
The throw keyword is used to throw an exception explicitly. Only object of Throwable class or its sub classes can be thrown. Program execution stops on encountering throw statement, and the closest catch statement is checked for matching type of exception.
finally clause:
A finally keyword is used to create a block of code that follows a try block. A finally block of code is always executed whether an exception has occurred or not. Using a finally block, it lets you run any cleanup type statements that you want to execute, no matter what happens in the protected code. A finally block appears at the end of catch block.
9. Explain static method in a class.
Ans. A static method is a method that belongs to a class rather than an instance of a class. This means you can call a static method without creating an object of the class. Static methods are sometimes called class methods.
You can access static methods from outside of the class in which they are defined. This is not possible with non-static methods.
Subclasses can override static methods, but non-static methods cannot.
Static methods are executed when an instance of the class is created, whereas non-static methods are not.
Static methods can be used to create utility classes that contain general-purpose methods.
You can use static methods to enforce encapsulation since they can only be called from within the class in which they are defined.
10. Define String class with all its method.
Ans. Java String class provides a lot of methods to perform operations on strings such as compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring() etc. The java.lang.String class implements Serializable, Comparable and CharSequence interfaces.
11. What hat is the difference between “Pass by value” and “Pass by reference” in java? Explain with proper example.
Ans.
Pass by Value: It is a process in which the function parameter values are copied to another variable and instead this object copied is passed. This is known as call by Value.
Pass by Reference: It is a process in which the actual copy of reference is passed to the function. This is called by Reference.
Example:
public class Mug {
private String contents;
public Mug(String contents) {
this.contents = contents;
}
public void setContents(String contents) {
this.contents = contents;
}
public String getContents(){
return contents;
}
}
public class Run {
public static void spill(Mug myMug) {
myMug.setContents("nothing");
}
public static void main(String args[]) {
Mug myMug = new Mug("tea"); // myMug contains "tea"
System.out.println(myMug.getContents());
spill(myMug); // myMug now contains "nothing"
System.out.println(myMug.getContents());
}
}
12. Explain “Array of Reference Type” using java code.
Ans. Java also allows the use of an interface name to specify a reference type. In addition, array types in Java are reference types because Java treats arrays as objects. The two main characteristics of objects in Java are that: Objects are always dynamically allocated.
1. Loops: for loop and for-each loop
int[] intArray = {2,5,46,12,34};
for(int i=0; i<intArray.length; i++){
System.out.print(intArray[i]);
// output: 25461234
}
for-each loop
int[] intArray = {2,5,46,12,34};
for(int i: intArray){
System.out.print(i);
// output: 25461234
}
2. Arrays.toString() method
String.valueOf() method :
int[] intArray = {2,5,46,12,34};
System.out.println(Arrays.toString(intArray));
// output: [2, 5, 46, 12, 34]
Object.toString() method:
public class Test {
public static void main(String[] args) {
Student[] students = {new Student("John"), new Student("Doe")};
System.out.println(Arrays.toString(students));
// output: [Student{name='John'}, Student{name='Doe'}]
}
}
class Student {
private String name;
public Student(String name){
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;}
@
Overridepublic String toString() {
return "Student{" + "name='" + name + '\'' + '}';
}
}
3. Arrays.deepToString() method
// creating multidimensional array
int[][] multiDimensionalArr = { {2,3}, {5,9} };
System.out.println(Arrays.deepToString(multiDimensionalArr));
// output: [[2, 3], [5, 9]]
4. Arrays.asList() method
Integer[] intArray = {2,5,46,12,34};
System.out.println(Arrays.asList(intArray));
// output: [2, 5, 46, 12, 34]
5. Java Iterator Interface
Integer[] intArray = {2,5,46,12,34};
// creating a List of Integer
List<Integer> list = Arrays.asList(intArray);
// creating an iterator of Integer List
Iterator<Integer> it = list.iterator();
// if List has elements to be iterated
while(it.hasNext()) {
System.out.print(it.next());
// output: 25461234
}
6. Java Stream API
Integer[] intArray = {2,5,46,12,34};
Arrays.stream(intArray).forEach(System.out::print);
// output: 25461234
13. Discuss final keyword used with variable, method and class.
Ans. Java final keyword is a non-access specifier that is used to restrict a class, variable, and method. If we initialize a variable with the final keyword, then we cannot modify its value.
If we declare a method as final, then it cannot be overridden by any subclasses. And, if we declare a class as final, we restrict the other classes to inherit or extend it.
In other words, the final classes can not be inherited by other classes.
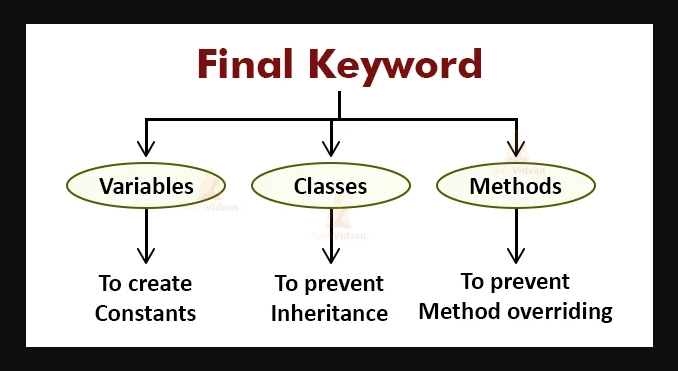
Final Variable in Java:
Once we declare a variable with the final keyword, we can’t change its value again. If we attempt to change the value of the final variable, then we will get a compilation error.
Final Method in Java:
As earlier, we discussed the Final Keyword and How to declare the Final Variable. We can declare Java methods as Final Method by adding the Final keyword before the method name.
Final Class in Java:
We can also declare a class with a final keyword in Java. When we declare a class as final, then we restrict other classes to inherit or extend it.
14. Describe default and parameterized constructor with example.
Ans.
Definition:
Default Constructor: A Constructor with no argument is called default Constructor
Parameterized Constructor: A Constructor with arguments is called Parameterized Constructor
Syntax:
//Default ConstructorClass ConstructorExample {
ConstructorExample (){
//Do something
}
}
//Parameterized ConstructorClass ConstructorExample {
<datatype> variableName;
ConstructorExample (<datatype> variableName){
this.variableName = variableName;
//Do something
}
}
Default and Parameterized Constructor Example Program :
public class DefaultAndParameterizedConstructor {
public static void main(String[] args) {
//Creating object of class using default constructor
DefaultConstructorExample object1 = new DefaultConstructorExample();
object1.doAddition();
//Creating object of class using parameterized constructor
ParameterizedConstructorExample object2 = new ParameterizedConstructorExample(100,200);
object2.doAddition();
}
}
class DefaultConstructorExample{
int num1 = 5, num2 = 10, result;
int doAddition(){
result = num1+num2;
System.out.println("This method is called using a default constructor");
return result;
}
}
class ParameterizedConstructorExample{
int num1, num2, result;
public ParameterizedConstructorExample(int num1, int num2) {
this.num1 = num1;
this.num2 = num2;
}
int doAddition(){
result = num1+num2;
System.out.println("This method is called using a parameterized constructor");
return result;
}
}
Sample Output :
This method is called using a default constructor
This method is called using a parameterized constructor
15. Discuss Accessibility of access modifiers in different condition.
Ans. There are two types of modifiers in Java: access modifiers and non-access modifiers.
The access modifiers in Java specifies the accessibility or scope of a field, method, constructor, or class. We can change the access level of fields, constructors, methods, and class by applying the access modifier on it.
There are four types of Java access modifiers:
Private: The access level of a private modifier is only within the class. It cannot be accessed from outside the class.
Default: The access level of a default modifier is only within the package. It cannot be accessed from outside the package. If you do not specify any access level, it will be the default.
Protected: The access level of a protected modifier is within the package and outside the package through child class. If you do not make the child class, it cannot be accessed from outside the package.
Public: The access level of a public modifier is everywhere. It can be accessed from within the class, outside the class, within the package and outside the package.
Examples :
1. Private:
class A{
private int data=40;
private void msg(){System.out.println("Hello java");}
}
public class Simple{
public static void main(String args[]){
A obj=new A();
System.out.println(obj.data);//Compile Time Error
obj.msg();//Compile Time Error
}
}
2. Default:
//save by A.java
package pack;
class A{
void msg(){System.out.println("Hello");}
}
//save by B.java
package mypack;
import pack.*;
class B{
public static void main(String args[]){
A obj = new A();//Compile Time Error
obj.msg();//Compile Time Error
}
}
3. Protected :
//save by A.java
package pack;
public class A{
protected void msg(){System.out.println("Hello");}
}
//save by B.java
package mypack;
import pack.*;
class B extends A{
public static void main(String args[]){
B obj = new B();
obj.msg();
}
}
4. Public :
//save by A.java
package pack;
public class A{
public void msg(){System.out.println("Hello");}
}
//save by B.java
package mypack;
import pack.*;
class B{
public static void main(String args[]){
A obj = new A();
obj.msg();
}
}
OUTPUT : Hello
Java Access Modifiers with Method Overriding
class A{
protected void msg(){System.out.println("Hello java");}
}
public class Simple extends A{
void msg(){System.out.println("Hello java");}//C.T.Error
public static void main(String args[]){
Simple obj=new Simple();
obj.msg();
}
}
16. Explain this keyword and its related concepts with example.
Ans.
Definition & Usage :
The this keyword refers to the current object in a method or constructor.
The most common use of the this keyword is to eliminate the confusion between class attributes and parameters with the same name (because a class attribute is shadowed by a method or constructor parameter). If you omit the keyword in the example above, the output would be "0" instead of "5".
this can also be used to:
Invoke current class constructor
Invoke current class method
Return the current class object
Pass an argument in the method call
Pass an argument in the constructor call
Example:
public class Main {
int x;
// Constructor with a parameter
public Main(int x) {
this.x = x;
}
// Call the constructor
public static void main(String[] args) {
Main myObj = new Main(5);
System.out.println("Value of x = " + myObj.x);
}
}
Output :
Value of x= 5
17. Differentiate Checked and Unchecked Exceptions.
Ans.
Checked Exceptions
They occur at compile time.
The compiler checks for a checked exception.
These exceptions can be handled at the compilation time.
It is a sub-class of the exception class.
The JVM requires that the exception be caught and handled.
Example of Checked exception- ‘File Not Found Exception’
Unchecked Exceptions
These exceptions occur at runtime.
The compiler doesn’t check for these kinds of exceptions.
These kinds of exceptions can’t be caught or handled during compilation time.
This is because the exceptions are generated due to the mistakes in the program.
These are not a part of the ‘Exception’ class since they are runtime exceptions.
The JVM doesn’t require the exception to be caught and handled.
Example of Unchecked Exceptions- ‘No Such Element Exception’.
Q. 4) 7-8 Marks Questions
1. Why Java is a platform independent language? How Java achieve this?
Ans. The platform can be defined as a distinct combination of hardware, operating system, and software that provides an environment to run programs.
Java is called Platform Independent because programs written in Java can be run on multiple platforms without re-writing them individually for a particular platform, i.e., Write Once Run Anywhere (WORA).
How Java Provides Platform Independence?
To understand how Java facilitates platform independence, let us differentiate between the compilation process of other programming languages, mainly C/C++, and that of Java.
The program written by the programmer, known as source code, is not understood by the computer. Source code is very similar to human language, consisting of words and phrases. It has to be converted into machine language code, which computers can easily understand and execute.
Machine language code is a set of instructions to be executed by the computer. The compiler does this conversion. The process of converting source code into machine code is called compilation. Machine Language Code is unique for different platforms.
Step-by-Step Execution of Java Programs:
Contrary to other compilers, the Java compiler doesn't produce native executable files or code for a particular platform. The compilation process in Java instead generates a special format called byte code. This generated file is also referred to as a .class file.
The Byte Code of Java is a set of machine instructions for a Java processor chip called Java Virtual Machine (JVM). Java Byte Code is very similar to machine language, but unlike machine language, Java byte code is absolutely the same on every platform. The byte code is not directly executable on any platform. Java code compiled into byte code still needs an interpreter to execute them on the platform.
Java Virtual Machine or JVM is a special Java Interpreter. Java Virtual Machine takes byte code as input. It interprets and executes the byte code and provides the output of the program.
Note: A compiler translates the entire program at once into machine-readable code. An Interpreter also converts the program to machine code, but it does so by translating it instruction-by-instruction or line-by-line.
The interpreter then converts the byte code into the native code. Native code is just like machine language code, which can be compiled to run with a particular processor and its set of instructions. Thus, native code can be executed by an individual operating system. Java Byte Code is platform-independent but natively executable code generated from byte code using an interpreter is highly platform-dependent and cannot run on other platforms.
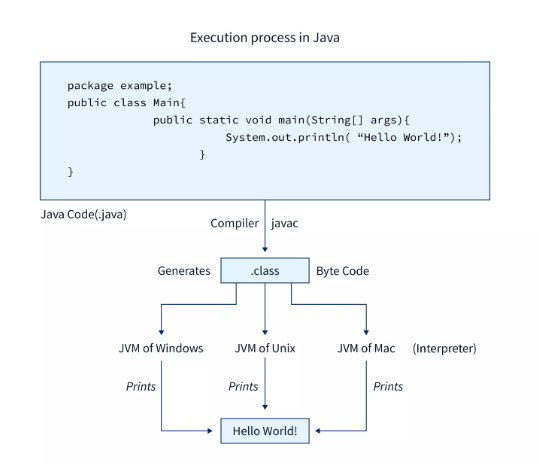
For example, source code written in windows OS is compiled by the javac compiler of windows, and the .class file (byte code) is created. JVM of Unix, MAC, or any other platform can interpret this byte code.
Hence, the JVM of that specific platform interprets the byte code and generates natively executable code for the platform. This native code is readily understood by the system and is executed, and it prints Hello World!
Using byte code, a natively executable code can be generated on any platform using a JVM interpreter, irrespective of the platform on which the byte code was created. But the natively executable code of one platform cannot be used for other platforms, i.e., the native code of Unix cannot run on Windows or MAC.
Note: Java code is both compiled and interpreted language. The source code written in Java is first compiled into bytecode. The Java Virtual Machine then interprets the generated bytecode for execution. However, the JVM also makes use of a Just in Time compiler during runtime to improve performances.
2. Explain Object oriented programming concepts in java.
Ans.
Object means a real-world entity such as a pen, chair, table, computer, watch, etc. Object-Oriented Programming is a methodology or paradigm to design a program using classes and objects. It simplifies software development and maintenance by providing some concepts:
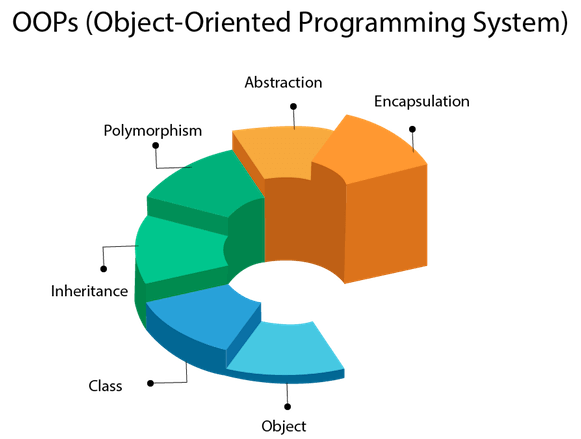
Object
Any entity that has state and behavior is known as an object. For example, a chair, pen, table, keyboard, bike, etc. It can be physical or logical.
An Object can be defined as an instance of a class. An object contains an address and takes up some space in memory. Objects can communicate without knowing the details of each other's data or code. The only necessary thing is the type of message accepted and the type of response returned by the objects.
Class
Collection of objects is called class. It is a logical entity.
A class can also be defined as a blueprint from which you can create an individual object. Class doesn't consume any space.
Inheritance
When one object acquires all the properties and behaviors of a parent object, it is known as inheritance. It provides code reusability. It is used to achieve runtime polymorphism.
Polymorphism
If one task is performed in different ways, it is known as polymorphism. For example: to convince the customer differently, to draw something, for example, shape, triangle, rectangle, etc.
In Java, we use method overloading and method overriding to achieve polymorphism.
Another example can be to speak something; for example, a cat speaks meow, dog barks woof, etc.
Abstraction
Hiding internal details and showing functionality is known as abstraction. For example phone call, we don't know the internal processing.
In Java, we use abstract class and interface to achieve abstraction.
Encapsulation
Binding (or wrapping) code and data together into a single unit are known as encapsulation. For example, a capsule, it is wrapped with different medicines.
A java class is the example of encapsulation. Java bean is the fully encapsulated class because all the data members are private here.
Coupling
Coupling refers to the knowledge or information or dependency of another class. It arises when classes are aware of each other. If a class has the details information of another class, there is strong coupling. In Java, we use private, protected, and public modifiers to display the visibility level of a class, method, and field. You can use interfaces for the weaker coupling because there is no concrete implementation.
Cohesion
Cohesion refers to the level of a component which performs a single well-defined task. A single well-defined task is done by a highly cohesive method. The weakly cohesive method will split the task into separate parts. The java.io package is a highly cohesive package because it has I/O related classes and interface. However, the java.util package is a weakly cohesive package because it has unrelated classes and interfaces.
Association
Association represents the relationship between the objects. Here, one object can be associated with one object or many objects. There can be four types of association between the objects:
One to One
One to Many
Many to One, and
Many to Many
Let's understand the relationship with real-time examples. For example, One country can have one prime minister (one to one), and a prime minister can have many ministers (one to many). Also, many MP's can have one prime minister (many to one), and many ministers can have many departments (many to many).
Association can be undirectional or bidirectional.
Aggregation
Aggregation is a way to achieve Association. Aggregation represents the relationship where one object contains other objects as a part of its state. It represents the weak relationship between objects. It is also termed as a has-a relationship in Java. Like, inheritance represents the is-a relationship. It is another way to reuse objects.
Composition
The composition is also a way to achieve Association. The composition represents the relationship where one object contains other objects as a part of its state. There is a strong relationship between the containing object and the dependent object. It is the state where containing objects do not have an independent existence. If you delete the parent object, all the child objects will be deleted automatically.
work in processing
Comments