Subject: Android Assignment-2
Unit: 2 Android Application Basics
BCA Semester: 5
1. Write a note on android life cycle.
Ans. Android Activity Lifecycle is controlled by 7 methods of android.app.Activity class. The android Activity is the subclass of ContextThemeWrapper class.
An activity is the single screen in android. It is like window or frame of Java.
By the help of activity, you can place all your UI components or widgets in a single screen.
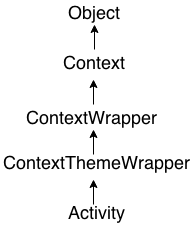
The 7 lifecycle method of Activity describes how activity will behave at different states.
Android Activity Lifecycle methods
Method | Description |
---|---|
onCreate | called when activity is first created. |
onStart | called when activity is becoming visible to the user. |
onResume | called when activity will start interacting with the user. |
onPause | called when activity is not visible to the user. |
onStop | called when activity is no longer visible to the user. |
onRestart | called after your activity is stopped, prior to start. |
onDestroy | called before the activity is destroyed. |
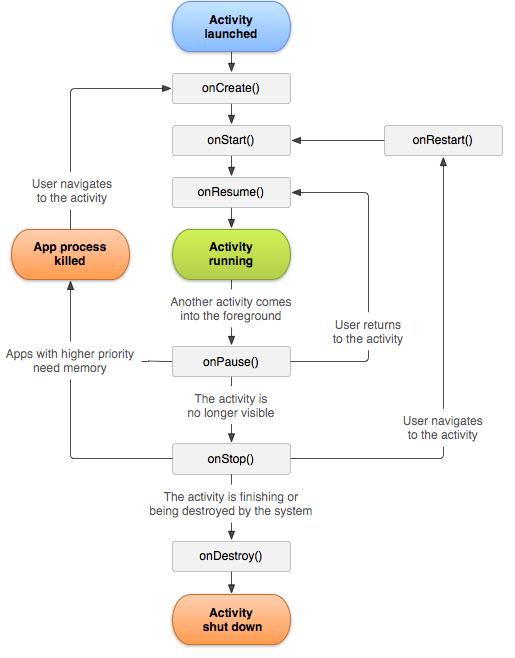
File: activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="example.javatpoint.com.activitylifecycle.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Android Activity Lifecycle Example
File: MainActivity.java
package example.javatpoint.com.activitylifecycle;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Log.d("lifecycle","onCreate invoked");
}
@Override
protected void onStart() {
super.onStart();
Log.d("lifecycle","onStart invoked");
}
@Override
protected void onResume() {
super.onResume();
Log.d("lifecycle","onResume invoked");
}
@Override
protected void onPause() {
super.onPause();
Log.d("lifecycle","onPause invoked");
}
@Override
protected void onStop() {
super.onStop();
Log.d("lifecycle","onStop invoked");
}
@Override
protected void onRestart() {
super.onRestart();
Log.d("lifecycle","onRestart invoked");
}
@Override
protected void onDestroy() {
super.onDestroy();
Log.d("lifecycle","onDestroy invoked");
}
}
Output:
You will not see any output on the emulator or device. You need to open logcat.
2. Explain Manifest File in detail.
Ans. Every project in Android includes a Manifest XML file, which is AndroidManifest.xml, located in the root directory of its project hierarchy. The manifest file is an important part of our app because it defines the structure and metadata of our application, its components, and its requirements. This file includes nodes for each of the Activities, Services, Content Providers, and Broadcast Receivers that make the application, and using Intent Filters and Permissions determines how they coordinate with each other and other applications.
The manifest file also specifies the application metadata, which includes its icon, version number, themes, etc., and additional top-level nodes can specify any required permissions, and unit tests, and define hardware, screen, or platform requirements. The manifest comprises a root manifest tag with a package attribute set to the project’s package. It should also include an xmls:android attribute that will supply several system attributes used within the file. We use the versionCode attribute is used to define the current application version in the form of an integer that increments itself with the iteration of the version due to update. Also, the versionName attribute is used to specify a public version that will be displayed to the users.
We can also specify whether our app should install on an SD card of the internal memory using the install Location attribute. A typical manifest file looks as:
XML Code:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.geeksforgeeks"
android:versionCode="1"
android:versionName="1.0"
android:installLocation="preferExternal">
<uses-sdk
android:minSdkVersion="18"
android:targetSdkVersion="27" />
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MyApplication"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
A manifest file includes the nodes that define the application components, security settings, test classes, and requirements that make up the application. Some of the manifest sub-node tags that are mainly used are:
1. manifest:
The main component of the AndroidManifest.xml file is known as manifest. Additionally, the packaging field describes the activity class’s package name. It must contain an <application> element with the xmlns:android and package attribute specified.
XML CODE:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.geeksforgeeks">
<!-- manifest nodes -->
<application>
</application>
</manifest>
2. uses-sdk :
It is used to define a minimum and maximum SDK version by means of an API Level integer that must be available on a device so that our application functions properly, and the target SDK for which it has been designed using a combination of minSdkVersion, maxSdkVersion, and targetSdkVersion attributes, respectively. It is contained within the <manifest> element.
XML Code:
<uses-sdk
android:minSdkVersion="18"
android:targetSdkVersion="27" />
3. uses-permission :
It outlines a system permission that must be granted by the user for the app to function properly and is contained within the <manifest> element. When an application is installed (on Android 5.1 and lower devices or Android 6.0 and higher), the user must grant the application permissions.
XML Code:
<uses-permission
android:name="android.permission.CAMERA"
android:maxSdkVersion="18" />
4. application :
A manifest can contain only one application node. It uses attributes to specify the metadata for your application (including its title, icon, and theme). During development, we should include a debuggable attribute set to true to enable debugging, then be sure to disable it for your release builds. The application node also acts as a container for the Activity, Service, Content Provider, and Broadcast Receiver nodes that specify the application components. The name of our custom application class can be specified using the android:name attribute.
XML Code:
<application
android:name=".GeeksForGeeks"
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@drawable/gfgIcon"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@android:style/Theme.Light"
android:debuggable="true"
tools:targetApi="31">
<!-- application nodes -->
</application>
5. uses-library :
It defines a shared library against which the application must be linked. This element instructs the system to add the library’s code to the package’s class loader. It is contained within the <application> element.
XML Code:
<uses-library
android:name="android.test.runner"
android:required="true" />
6. activity :
The Activity sub-element of an application refers to an activity that needs to be specified in the AndroidManifest.xml file. It has various characteristics, like label, name, theme, launchMode, and others. In the manifest file, all elements must be represented by <activity>. Any activity that is not declared there won’t run and won’t be visible to the system. It is contained within the <application> element.
XML Code:
<activity
android:name=".MainActivity"
android:exported="true">
</activity>
7. intent-filter :
It is the sub-element of activity that specifies the type of intent to which the activity, service, or broadcast receiver can send a response. It allows the component to receive intents of a certain type while filtering out those that are not useful for the component. The intent filter must contain at least one <action> element.
XML Code:
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
8. action :
It adds an action for the intent-filter. It is contained within the <intent-filter> element.
XML Code:
<action android:name="android.intent.action.MAIN" />
9. category :
It adds a category name to an intent-filter. It is contained within the <intent-filter> element.
XML Code:
<category android:name="android.intent.category.LAUNCHER" />
10. uses-configuration :
The uses-configuration components are used to specify the combination of input mechanisms that are supported by our application. It is useful for games that require particular input controls.
XML Code:
<uses-configuration
android:reqTouchScreen=”finger”
android:reqNavigation=”trackball”
android:reqHardKeyboard=”true”
android:reqKeyboardType=”qwerty”/>
<uses-configuration
android:reqTouchScreen=”finger”
android:reqNavigation=”trackball”
android:reqHardKeyboard=”true”
android:reqKeyboardType=”twelvekey”/>
11. uses-features :
It is used to specify which hardware features your application requires. This will prevent our application from being installed on a device that does not include a required piece of hardware such as NFC hardware, as follows:
XML Code:
<uses-feature android:name=”android.hardware.nfc” />
12. permission :
It is used to create permissions to restrict access to shared application components. We can also use the existing platform permissions for this purpose or define your own permissions in the manifest.
XML Code:
<permission
android: name=”com.paad.DETONATE_DEVICE”
android:protectionLevel=“dangerous”
android:label=”Self Destruct”
android:description=”@string/detonate_description”>
</permission>
3. Explain any 10 different types of resources file andriod.
Ans.
1. Bool :
A Boolean value defined in XML.
file location:res/values/filename.xml
The filename is arbitrary. The <bool> element's name is used as the resource ID.
resource reference:
In Java: R.bool.bool_name
In XML: @[package:]bool/bool_name
Syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<bool
name="bool_name"
>[true | false]</bool>
</resources>
2. Color :
A color value defined in XML. The color is specified using an RGB value and alpha channel. You can use a color resource any place that accepts a hexadecimal color value. You can also use a color resource when a drawable resource is expected in XML, such as android:drawable="@color/green".
file location: res/values/colors.xml
The filename is arbitrary. The <color> element's name is used as the resource ID.
resource reference:
In Java: R.color.color_name
In XML: @[package:]color/color_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color
name="color_name"
>hex_color</color>
</resources>
3. Dimension :
A dimension value defined in XML. A dimension is specified with a number followed by a unit of measure, such as 10px, 2in, or 5sp. The following units of measure are supported by Android:
dp : Density-independent pixels: an abstract unit that is based on the physical density of the screen. These units are relative to a 160 dpi (dots per inch) screen, on which 1 dp is roughly equal to 1 px. When running on a higher density screen, the number of pixels used to draw 1 dp is scaled up by a factor appropriate for the screen's dpi.
sp: Scale-independent Pixels - This is like the dp unit, but it is also scaled by the user's font size preference. It is recommend you use this unit when specifying font sizes, so they will be adjusted for both the screen density and the user's preference.
pt: Points: 1/72 of an inch based on the physical size of the screen, assuming a 72 dpi density screen.
px: Pixels: corresponds to actual pixels on the screen. We don't recommend using this unit, because the actual representation can vary across devices. Different devices can have a different number of pixels per inch and might have more or fewer total pixels available on the screen.
mm: Millimeters: based on the physical size of the screen.
in: Inches: based on the physical size of the screen.
file location: res/values/filename.xml
The filename is arbitrary. The <dimen> element's name is used as the resource ID.
resource reference:
In Java: R.dimen.dimension_name
In XML: @[package:]dimen/dimension_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen
name="dimension_name"
>dimension</dimen>
</resources>
4. ID :
A unique resource ID defined in XML. Using the name you provide in the <item> element, the Android developer tools create a unique integer in your project's R.java class, which you can use as an identifier for an application resources, such as a View in your UI layout, or a unique integer for use in your application code, such as an ID for a dialog or a result code.
file location:res/values/filename.xml
The filename is arbitrary.
resource reference:
In Java: R.id.name
In XML: @[package:]id/name
syntax:
5. Integer :
An integer defined in XML.
file location: res/values/filename.xml
The filename is arbitrary. The <integer> element's name is used as the resource ID.
resource reference:
In Java: R.integer.integer_name
In XML: @[package:]integer/integer_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<integer
name="integer_name"
>integer</integer>
</resources>
6. Integer array :
An array of integers defined in XML.
file location: res/values/filename.xml
The filename is arbitrary. The <integer-array> element's name is used as the resource ID.
compiled resource datatype: Resource pointer to an array of integers.
resource reference:
In Java: R.array.integer_array_name
In XML: @[package:]array/integer_array_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<integer-array
name="integer_array_name">
<item
>integer</item>
</integer-array>
</resources>
7. Typed array :
A TypedArray defined in XML. You can use this to create an array of other resources, such as drawables. The array isn't required to be homogeneous, so you can create an array of mixed resource types, but be aware of what and where the data types are in the array so that you can properly obtain each item with the TypedArray class's get...() methods.
file location: res/values/filename.xml
The filename is arbitrary. The <array> element's name is used as the resource ID.
compiled resource datatype: Resource pointer to a TypedArray.
resource reference:
In Java: R.array.array_name
In XML: @[package:]array/array_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<array
name="integer_array_name">
<item>resource</item>
</array>
</resources>
8. Layout resource:
A layout resource defines the architecture for the UI in an Activity or a component of a UI. file location: res/layout/filename.xml The filename is used as the resource ID.
compiled resource datatype: Resource pointer to a View (or subclass)
resource reference:
In Java: R.layout.filename In XML: @[package:]layout/filename
syntax:
<?xml version="1.0" encoding="utf-8"?>
<ViewGroup
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@[+][package:]id/resource_name"
android:layout_height=["dimension" | "match_parent" | "wrap_content"]
android:layout_width=["dimension" | "match_parent" | "wrap_content"]
[ViewGroup-specific attributes] >
<View
android:id="@[+][package:]id/resource_name"
android:layout_height=["dimension" | "match_parent" | "wrap_content"]
android:layout_width=["dimension" | "match_parent" | "wrap_content"]
[View-specific attributes] >
<requestFocus/>
</View>
<ViewGroup >
<View />
</ViewGroup>
<include layout="@layout/layout_resource"/>
</ViewGroup>
9. String:
A single string that can be referenced from the application or from other resource files (such as an XML layout).
file location: res/values/filename.xml
The filename is arbitrary. The <string> element's name is used as the resource ID.
compiled resource datatype: Resource pointer to a String.
resource reference:
In Java: R.string.string_name
In XML: @string/string_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string
name="string_name"
>text_string</string>
</resources>
10. Style resource :
A style resource defines the format and look for a UI. A style can be applied to an individual View (from within a layout file) or to an entire Activity or application (from within the manifest file).
file location: res/values/filename.xml
The filename is arbitrary. The element's name will be used as the resource ID.
resource reference:
In XML: @[package:]style/style_name
syntax:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style
name="style_name"
parent="@[package:]style/style_to_inherit">
<item
name="[package:]style_property_name"
>style_value</item>
</style>
</resources>
4. Explain Menu and Scroll View.
Ans.
Android Menus:
In android, Menu is an important part of the UI component which is used to provide some common functionality around the application. With the help of menu, users can experience a smooth and consistent experience throughout the application. In order to use menu, we should define it in a separate XML file and use that file in our application based on our requirements. Also, we can use menu APIs to represent user actions and other options in our android application activities.
How to define Menu in XML File?
Android Studio provides a standard XML format for the type of menus to define menu items. We can simply define the menu and all its items in XML menu resource instead of building the menu in the code and also load menu resource as menu object in the activity or fragment used in our android application. Here, we should create a new folder menu inside of our project directory (res/menu) to define the menu and also add a new XML file to build the menu with the following elements. Below is the example of defining a menu in the XML file (menu_example.xml).
Way to create menu directory and menu resource file:
To create the menu directory just right-click on res folder and navigate to res->New->Android Resource Directory. Give resource directory name as menu and resource type also menu. one directory will be created under res folder.
To create xml resource file simply right-click on menu folder and navigate to New->Menu Resource File. Give name of file as menu_example. One menu_example.xml file will be created under menu folder.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http:// schemas.android.com/apk/res/android">
<item android:id="@+id/mail"
android:icon="@drawable/ic_mail"
android:title="@string/mail" />
<item android:id="@+id/upload"
android:icon="@drawable/ic_upload"
android:title="@string/upload"
android:showAsAction="ifRoom" />
<item android:id="@+id/share"
android:icon="@drawable/ic_share"
android:title="@string/share" />
</menu>
<menu> It is the root element that helps in defining Menu in XML file and it also holds multiple elements.
<item> It is used to create a single item in the menu. It also contains nested <menu> element in order to create a submenu.
<group> It is optional and invisible for <item> elements to categorize the menu items so they can share properties like active state, and visibility.
activity_main.xml :
If we want to add a submenu in menu item, then we need to add a <menu> element as the child of an <item>. Below is the example of defining a submenu in a menu item.
XML Code:
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http:// schemas.android.com/apk/res/android">
<item android:id="@+id/file"
android:title="@string/file" >
<!-- "file" submenu -->
<menu>
<item android:id="@+id/create_new"
android:title="@string/create_new" />
<item android:id="@+id/open"
android:title="@string/open" />
</menu>
</item>
</menu>
Android Different Types of Menus :
In android, we have three types of Menus available to define a set of options and actions in our android applications. The Menus in android applications are the following:
Android Options Menu
Android Context Menu
Android Popup Menu
Android Options Menu is a primary collection of menu items in an android application and is useful for actions that have a global impact on the searching application. Android Context Menu is a floating menu that only appears when the user clicks for a long time on an element and is useful for elements that affect the selected content or context frame. Android Popup Menu displays a list of items in a vertical list which presents the view that invoked the menu and is useful to provide an overflow of actions related to specific content.
Android Scroll View :
ScrollView is a view group that we can use to create vertically scrollable views. There is only one direct child in a scroll view. Because a ScrollView can only scroll vertically, you'll need to use a Horizontal Scroll View to create a horizontally scrollable view.
The ScrollView tag is used to create a Scroll View in an android application.
For example:
<ScrollView
android:id="@+id/scrollView2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/scroll_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:text="@string/scroll_text" />
</ScrollView>
In the above example, Scroll View has one child, which is a TextView widget. Here the android:orientation of the Text View widget is set to vertical. It defines whether to display child views in a row or column.
Attributes of Scroll View :
Following are some of the important attributes of a Scroll View:
android:id: A Unique id to identify any widget
android:layout_width: It defines the width of the layout
android:layout_height: It sets the layout's height
android:layout_marginStart: The amount of space needed at the start of the widget
android:layout_marginEnd: It represents the amount of space needed on the End of the widget
android:inputType: This allows you to define the input method's behaviour
android:text: Specifies the default text displayed in the field
app:layout_constraintTop_toTopOf: Align the desired view's top with the top of another
Horizontal Scroll View :
Horizontal Scroll View is used to add horizontal scroll ability in an android application. We can use the Horizontal Scroll View tag to create the same.
5. Working with permissions android explain?
Ans.
Restricted data, such as system state and users' contact information
Restricted actions, such as connecting to a paired device and recording audio
Workflow for using permissions :
If your app offers functionality that might require access to restricted data or restricted actions, determine whether you can get the information or perform the actions without needing to declare permissions. You can fulfill many use cases in your app, such as taking photos, pausing media playback, and displaying relevant ads, without needing to declare any permissions.
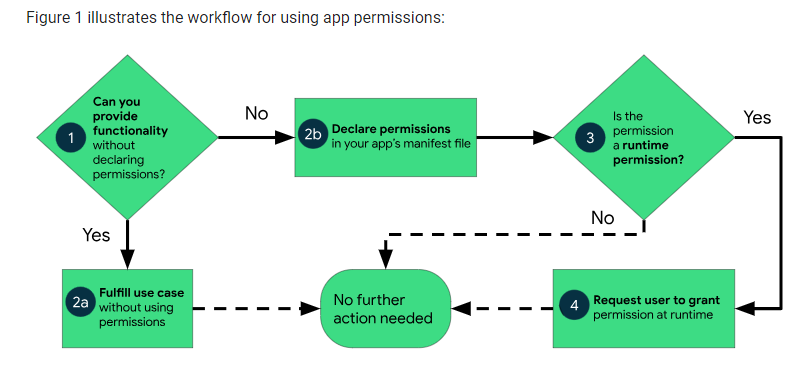
Types of permissions :
Android categorizes permissions into different types, including install-time permissions, runtime permissions, and special permissions. Each permission's type indicates the scope of restricted data that your app can access, and the scope of restricted actions that your app can perform, when the system grants your app that permission. The protection level for each permission is based on its type and is shown on the permissions API reference page.
Install-time permissions:
Install-time permissions give your app limited access to restricted data or let your app perform restricted actions that minimally affect the system or other apps. When you declare install-time permissions in your app, an app store presents an install-time permission notice to the user when they view an app's details page, as shown in figure 2. The system automatically grants your app the permissions when the user installs your app.
Normal permissions:
These permissions allow access to data and actions that extend beyond your app's sandbox but present very little risk to the user's privacy and the operation of other apps.
The system assigns the normal protection level to normal permissions.
Signature permissions:
The system grants a signature permission to an app only when the app is signed by the same certificate as the app or the OS that defines the permission.
Runtime permissions:
Runtime permissions, also known as dangerous permissions, give your app additional access to restricted data or let your app perform restricted actions that more substantially affect the system and other apps. Therefore, you need to request runtime permissions in your app before you can access the restricted data or perform restricted actions. Don't assume that these permissions have been previously granted—check them and, if needed, request them before each access.
Special permissions:
Special permissions correspond to particular app operations. Only the platform and OEMs can define special permissions. Additionally, the platform and OEMs usually define special permissions when they want to protect access to particularly powerful actions, such as drawing over other apps.
Permission groups:
Permissions can belong to permission groups. Permission groups consist of a set of logically related permissions. For example, permissions to send and receive SMS messages might belong to the same group, as they both relate to the application's interaction with SMS.
nice work
.